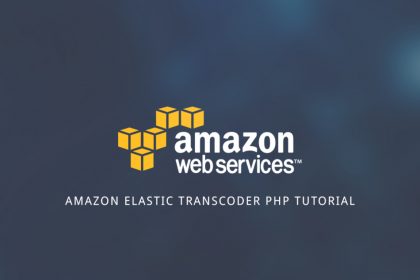
Many of us are using AWS—But sometimes problems arise when reading hundreds of lines of argument to make a simple process with Amazon Elastic Transcoder. For this reason I created a short tutorial about transcoder in order to have a quick review of what you will need for simple operations—I hope you’ll get some use out of it. If you do, let me know!
The AWS PHP SDK
So to start the process first you will need to implement the AWS SDK.
You can download the SDK from here: AWS SDK
Or if you are using composer you can simply add this to your composer.json
"require":
{
"aws/aws-sdk-php": "2.7.*@dev"
}
The AWS Transcoder Configuration
I’m not going to explain in this tutorial how to setup the transcoding pipeline, but you can check more information about AWS console configuration in this tutorial.

The AWS instance and functions
For start, we will need to create a new instance of ElasticTranscoderClient. This will help us create new jobs and read other jobs that we created.
use Aws\ElasticTranscoder\ElasticTranscoderClient;
$elasticTranscoder = ElasticTranscoderClient::factory(array(
'credentials' => array(
'key' => 'your key',
'secret' => 'your secret',
),
'region' => 'us-east-1', // dont forget to set the region
));
With this code you will have an amazon instance that will try to connect with your AWS console IAM user generated from console
Now with the instance already created, we will try to create a new job with the code written below.
$job = $elasticTranscoder->createJob(array(
'PipelineId' => '',
'OutputKeyPrefix' => 'Output prefix added to the file',
'Input' => array(
'Key' => 'the path from bucket to the file, with file name',
'FrameRate' => 'auto',
'Resolution' => 'auto',
'AspectRatio' => 'auto',
'Interlaced' => 'auto',
'Container' => 'auto',
),
'Outputs' => array(
array(
'Key' => 'myOutput.mp4',
'Rotate' => 'auto',
'PresetId' => '',
),
),
));
// get the job data as array
$jobData = $job->get('Job');
// you can save the job ID somewhere, so you can check
// the status from time to time.
$jobId = $jobData['Id'];
This will create a transcoder job. Now to be more clear, I will try to explain all the data that is more important on your transcoder configuration
Pipeline Id – The id of the generated pipeline in AWS console
Output key prefix – The output prefix is added before the output file name if you add example myprefix/ this will create a subfolder in bucket with the folder name myprefix
Input[Key] – The input key is the path to the file on S3 like myprefix/oldVideo.mp4
Outputs[PresetId] – The preset id is a convertor type what you will use for the new transcoded video, you can find all the preset id’s in AWS console Elastic Transcoder
Check job status
You probably want to notify someone when the transcoding is done—you could create an callback, but that would require your application to be accessible at one point from the outside. The other method is polling the status until the jobs status changes to success or error.
$jobStatusRequest = $elasticTranscoder->readJob(array('Id' => $jobId));
$jobData = $jobStatusRequest->get('Job');
if ($jobData['Status'] !== 'progressing'
&& $jobData['Status'] !== 'submitted')
{
echo $jobData['Status'];
}
Thank you for reading my tutorial and hope that it helps,
If you have some questions don’t hesitate to comment.
***
GloobusTech is a series of technical articles posted by the team members of Gloobus. If you would like us to touch on some specific technical issues, please get in touch.